Nowadays camera become one the major features that lot of apps provides. You can see the camera feature excessively used in apps in the area of food, social networking, video sharing apps. Integrating camera in your app is very simple task if you are not expecting a custom UI.
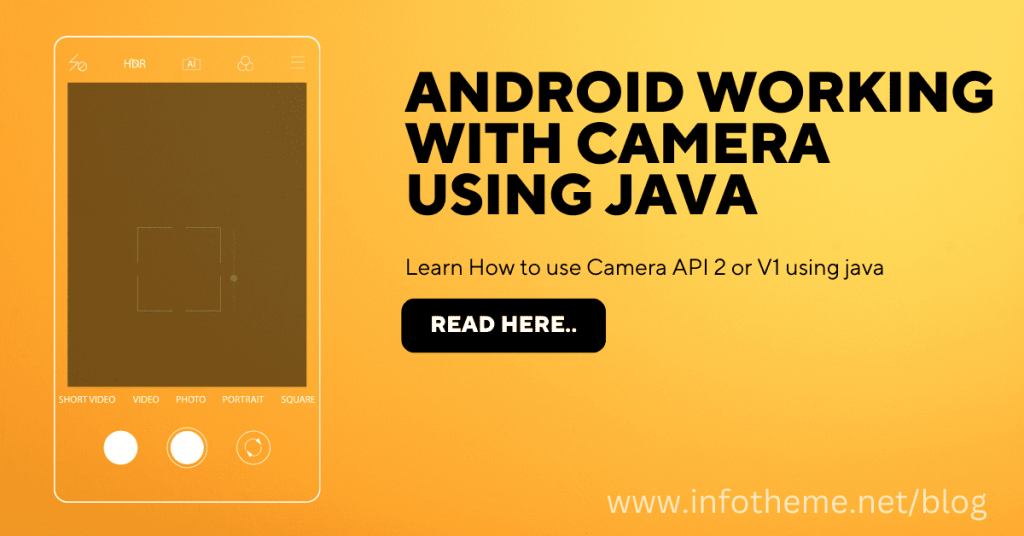
In this article we are going to see how to integrate the basic camera feature that takes pictures or record video through your app.
1. Android Camera API
There are two ways to integrate the camera module.
- Using In-built Camera App:
- Writing Custom Camera App:
Below is the demo app that we gonna build in this article. The UI is very minimal with two buttons and an area to preview the captured image or video.

Now let’s start by creating a new project in Android Studio.
2. Creating New Project
1. Create a new project in Android Studio from File ⇒ New Project and select Basic Activity from templates.
2. Open app/build.gradle and add Dexter dependency to request the runtime permissions.
app/build.gradle dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) // ... // dexter runtime permissions implementation 'com.karumi:dexter:4.2.0' }
3. Add the below resources to respective strings.xml, colors.xml and dimens.xml
strings.xml <resources> <string name="app_name">Android Camera</string> <string name="action_settings">Settings</string> <string name="preview_description">Image and Video preview will appear here</string> <string name="btn_take_picture">Take Picture</string> <string name="btn_record_video">Record Video</string> </resources>
colors.xml <?xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#1fb49c</color> <color name="colorPrimaryDark">#1fb49c</color> <color name="colorAccent">#FF4081</color> </resources>
dimens.xml <resources> <dimen name="activity_margin">16dp</dimen> <dimen name="dimen_8">8dp</dimen> </resources>
4. Under res, create a new folder named xml. Inside xml folder, create a new xml file name file_paths.xml
file_paths.xml <?xml version="1.0" encoding="utf-8"?> <paths xmlns:android="http://schemas.android.com/apk/res/android"> <external-path name="external_files" path="."/> </paths>
5. Open AndroidManifest.xml and add CAMERA, WRITE_EXTERNAL_STORAGE and RECORD_AUDIO permissions.
Here we also added camera feature that defines the rules to list the app on Playstore. If we keep android:required=”true”, Google Playstore won’t let the users to install the app on the devices that doesn’t have camera feature. Incase of false, the app will be listed but camera feature is optional.
We also need <provider> tag to prepare the file paths properly across all the android platforms.
<uses-feature android:name="android.hardware.camera" android:required="false" />
AndroidManifest.xml <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="net.infotheme.androidcamera"> <uses-permission android:name="android.permission.CAMERA" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.RECORD_AUDIO" /> <uses-feature android:name="android.hardware.camera" android:required="false" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity" android:label="@string/app_name" android:theme="@style/AppTheme.NoActionBar"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <provider android:name="android.support.v4.content.FileProvider" android:authorities="${applicationId}.provider" android:exported="false" android:grantUriPermissions="true"> <meta-data android:name="android.support.FILE_PROVIDER_PATHS" android:resource="@xml/file_paths" /> </provider> </application> </manifest>
6. Create a class named CameraUtils.java and add the below methods. This is a helper class that provides necessary methods that we need in our camera module.
- refreshGallery() – Refreshes the image gallery after taking a new picture or video so that they will be instantly available in the gallery. In older android devices, gallery won’t be refreshed until the device is rebooted.
- checkPermissions() – Checks whether all the permissions are granted or not. This would be called before requesting the camera.
- getOutputMediaFile() – Create a new file in gallery and returns the file. This reference will be passed to camera intent so that newly taken image / video will be stored in this location.
- optimizeBitmap() – Compresses the bitmap before displaying it on UI to avoid the OutOfMemory exceptions.
CameraUtils.java package net.infotheme.androidcamera; import android.Manifest; import android.content.Context; import android.content.Intent; import android.content.pm.PackageManager; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.media.MediaScannerConnection; import android.net.Uri; import android.os.Environment; import android.provider.Settings; import android.support.v4.app.ActivityCompat; import android.support.v4.content.FileProvider; import android.util.Log; import java.io.File; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Locale; public class CameraUtils { /** * Refreshes gallery on adding new image/video. Gallery won't be refreshed * on older devices until device is rebooted */ public static void refreshGallery(Context context, String filePath) { // ScanFile so it will be appeared on Gallery MediaScannerConnection.scanFile(context, new String[]{filePath}, null, new MediaScannerConnection.OnScanCompletedListener() { public void onScanCompleted(String path, Uri uri) { } }); } public static boolean checkPermissions(Context context) { return ActivityCompat.checkSelfPermission(context, Manifest.permission.CAMERA) == PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(context, Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(context, Manifest.permission.RECORD_AUDIO) == PackageManager.PERMISSION_GRANTED; } /** * Downsizing the bitmap to avoid OutOfMemory exceptions */ public static Bitmap optimizeBitmap(int sampleSize, String filePath) { // bitmap factory BitmapFactory.Options options = new BitmapFactory.Options(); // downsizing image as it throws OutOfMemory Exception for larger // images options.inSampleSize = sampleSize; return BitmapFactory.decodeFile(filePath, options); } /** * Checks whether device has camera or not. This method not necessary if * android:required="true" is used in manifest file */ public static boolean isDeviceSupportCamera(Context context) { if (context.getPackageManager().hasSystemFeature( PackageManager.FEATURE_CAMERA)) { // this device has a camera return true; } else { // no camera on this device return false; } } /** * Open device app settings to allow user to enable permissions */ public static void openSettings(Context context) { Intent intent = new Intent(); intent.setAction(Settings.ACTION_APPLICATION_DETAILS_SETTINGS); intent.setData(Uri.fromParts("package", BuildConfig.APPLICATION_ID, null)); intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); context.startActivity(intent); } public static Uri getOutputMediaFileUri(Context context, File file) { return FileProvider.getUriForFile(context, context.getPackageName() + ".provider", file); } /** * Creates and returns the image or video file before opening the camera */ public static File getOutputMediaFile(int type) { // External sdcard location File mediaStorageDir = new File( Environment .getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES), MainActivity.GALLERY_DIRECTORY_NAME); // Create the storage directory if it does not exist if (!mediaStorageDir.exists()) { if (!mediaStorageDir.mkdirs()) { Log.e(MainActivity.GALLERY_DIRECTORY_NAME, "Oops! Failed create " + MainActivity.GALLERY_DIRECTORY_NAME + " directory"); return null; } } // Preparing media file naming convention // adds timestamp String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.getDefault()).format(new Date()); File mediaFile; if (type == MainActivity.MEDIA_TYPE_IMAGE) { mediaFile = new File(mediaStorageDir.getPath() + File.separator + "IMG_" + timeStamp + "." + MainActivity.IMAGE_EXTENSION); } else if (type == MainActivity.MEDIA_TYPE_VIDEO) { mediaFile = new File(mediaStorageDir.getPath() + File.separator + "VID_" + timeStamp + "." + MainActivity.VIDEO_EXTENSION); } else { return null; } return mediaFile; } }
7. Now open the layout files of main activity i.e activity_main.xml and content_main.xml and add the below layout code. Here we are defining couple of Buttons, ImageView and VideoView to preview the captured media.
activity_main.xml <?xml version="1.0" encoding="utf-8"?> <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <android.support.design.widget.AppBarLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:theme="@style/AppTheme.AppBarOverlay"> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" app:popupTheme="@style/AppTheme.PopupOverlay" /> </android.support.design.widget.AppBarLayout> <include layout="@layout/content_main" /> </android.support.design.widget.CoordinatorLayout>
content_main.xml <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:padding="@dimen/activity_margin" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context=".MainActivity" tools:showIn="@layout/activity_main"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:layout_marginBottom="@dimen/activity_margin" android:layout_weight="1" android:orientation="vertical"> <TextView android:id="@+id/txt_desc" android:layout_width="fill_parent" android:layout_height="match_parent" android:gravity="center" android:padding="10dp" android:text="@string/preview_description" android:textSize="15dp" /> <!-- To display picture taken --> <ImageView android:id="@+id/imgPreview" android:layout_width="match_parent" android:layout_height="match_parent" android:scaleType="centerCrop" android:visibility="gone" /> <!-- To preview video recorded --> <VideoView android:id="@+id/videoPreview" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center_horizontal" android:visibility="gone" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:weightSum="2"> <!-- Capture picture button --> <Button android:id="@+id/btnCapturePicture" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginRight="@dimen/dimen_8" android:layout_weight="1" android:background="@color/colorPrimary" android:foreground="?attr/selectableItemBackground" android:text="@string/btn_take_picture" android:textColor="@android:color/white" /> <!-- Record video button --> <Button android:id="@+id/btnRecordVideo" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/dimen_8" android:layout_weight="1" android:background="@color/colorPrimary" android:foreground="?attr/selectableItemBackground" android:text="@string/btn_record_video" android:textColor="@android:color/white" /> </LinearLayout> </LinearLayout>
8. Finally open MainActivity.java and modify the code as shown below.
- In onCreate(), the availability of the camera is checked using isDeviceSupportCamera() method and activity is closed if the camera is absent on the device.
- On button click, checkPermissions() method is used the check whether required permissions are granted or not. If not granted, requestCameraPermission() method shows the permissions dialog to user.
- captureImage() open the camera app to capture the image.
- captureVideo() opens the camera app to record the video.
- Once the media is captured, the image or video will be saved to gallery. previewCapturedImage() and previewVideo() renders the captured image / video on the screen.
- showPermissionsAlert() – Shows an alert dialog propting user to enable the permissions in app settings incase they are denied permanently.
MainActivity.java package net.infotheme.androidcamera; import android.Manifest; import android.content.DialogInterface; import android.content.Intent; import android.graphics.Bitmap; import android.net.Uri; import android.os.Bundle; import android.provider.MediaStore; import android.support.v7.app.AlertDialog; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.Toolbar; import android.text.TextUtils; import android.view.View; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView; import android.widget.Toast; import android.widget.VideoView; import com.karumi.dexter.Dexter; import com.karumi.dexter.MultiplePermissionsReport; import com.karumi.dexter.PermissionToken; import com.karumi.dexter.listener.PermissionRequest; import com.karumi.dexter.listener.multi.MultiplePermissionsListener; import java.io.File; import java.util.List; public class MainActivity extends AppCompatActivity { // Activity request codes private static final int CAMERA_CAPTURE_IMAGE_REQUEST_CODE = 100; private static final int CAMERA_CAPTURE_VIDEO_REQUEST_CODE = 200; // key to store image path in savedInstance state public static final String KEY_IMAGE_STORAGE_PATH = "image_path"; public static final int MEDIA_TYPE_IMAGE = 1; public static final int MEDIA_TYPE_VIDEO = 2; // Bitmap sampling size public static final int BITMAP_SAMPLE_SIZE = 8; // Gallery directory name to store the images or videos public static final String GALLERY_DIRECTORY_NAME = "Hello Camera"; // Image and Video file extensions public static final String IMAGE_EXTENSION = "jpg"; public static final String VIDEO_EXTENSION = "mp4"; private static String imageStoragePath; private TextView txtDescription; private ImageView imgPreview; private VideoView videoPreview; private Button btnCapturePicture, btnRecordVideo; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = findViewById(R.id.toolbar); setSupportActionBar(toolbar); // Checking availability of the camera if (!CameraUtils.isDeviceSupportCamera(getApplicationContext())) { Toast.makeText(getApplicationContext(), "Sorry! Your device doesn't support camera", Toast.LENGTH_LONG).show(); // will close the app if the device doesn't have camera finish(); } txtDescription = findViewById(R.id.txt_desc); imgPreview = findViewById(R.id.imgPreview); videoPreview = findViewById(R.id.videoPreview); btnCapturePicture = findViewById(R.id.btnCapturePicture); btnRecordVideo = findViewById(R.id.btnRecordVideo); /** * Capture image on button click */ btnCapturePicture.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (CameraUtils.checkPermissions(getApplicationContext())) { captureImage(); } else { requestCameraPermission(MEDIA_TYPE_IMAGE); } } }); /** * Record video on button click */ btnRecordVideo.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (CameraUtils.checkPermissions(getApplicationContext())) { captureVideo(); } else { requestCameraPermission(MEDIA_TYPE_VIDEO); } } }); // restoring storage image path from saved instance state // otherwise the path will be null on device rotation restoreFromBundle(savedInstanceState); } /** * Restoring store image path from saved instance state */ private void restoreFromBundle(Bundle savedInstanceState) { if (savedInstanceState != null) { if (savedInstanceState.containsKey(KEY_IMAGE_STORAGE_PATH)) { imageStoragePath = savedInstanceState.getString(KEY_IMAGE_STORAGE_PATH); if (!TextUtils.isEmpty(imageStoragePath)) { if (imageStoragePath.substring(imageStoragePath.lastIndexOf(".")).equals("." + IMAGE_EXTENSION)) { previewCapturedImage(); } else if (imageStoragePath.substring(imageStoragePath.lastIndexOf(".")).equals("." + VIDEO_EXTENSION)) { previewVideo(); } } } } } /** * Requesting permissions using Dexter library */ private void requestCameraPermission(final int type) { Dexter.withActivity(this) .withPermissions(Manifest.permission.CAMERA, Manifest.permission.WRITE_EXTERNAL_STORAGE, Manifest.permission.RECORD_AUDIO) .withListener(new MultiplePermissionsListener() { @Override public void onPermissionsChecked(MultiplePermissionsReport report) { if (report.areAllPermissionsGranted()) { if (type == MEDIA_TYPE_IMAGE) { // capture picture captureImage(); } else { captureVideo(); } } else if (report.isAnyPermissionPermanentlyDenied()) { showPermissionsAlert(); } } @Override public void onPermissionRationaleShouldBeShown(List<PermissionRequest> permissions, PermissionToken token) { token.continuePermissionRequest(); } }).check(); } /** * Capturing Camera Image will launch camera app requested image capture */ private void captureImage() { Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); File file = CameraUtils.getOutputMediaFile(MEDIA_TYPE_IMAGE); if (file != null) { imageStoragePath = file.getAbsolutePath(); } Uri fileUri = CameraUtils.getOutputMediaFileUri(getApplicationContext(), file); intent.putExtra(MediaStore.EXTRA_OUTPUT, fileUri); // start the image capture Intent startActivityForResult(intent, CAMERA_CAPTURE_IMAGE_REQUEST_CODE); } /** * Saving stored image path to saved instance state */ @Override protected void onSaveInstanceState(Bundle outState) { super.onSaveInstanceState(outState); // save file url in bundle as it will be null on screen orientation // changes outState.putString(KEY_IMAGE_STORAGE_PATH, imageStoragePath); } /** * Restoring image path from saved instance state */ @Override protected void onRestoreInstanceState(Bundle savedInstanceState) { super.onRestoreInstanceState(savedInstanceState); // get the file url imageStoragePath = savedInstanceState.getString(KEY_IMAGE_STORAGE_PATH); } /** * Launching camera app to record video */ private void captureVideo() { Intent intent = new Intent(MediaStore.ACTION_VIDEO_CAPTURE); File file = CameraUtils.getOutputMediaFile(MEDIA_TYPE_VIDEO); if (file != null) { imageStoragePath = file.getAbsolutePath(); } Uri fileUri = CameraUtils.getOutputMediaFileUri(getApplicationContext(), file); // set video quality intent.putExtra(MediaStore.EXTRA_VIDEO_QUALITY, 1); intent.putExtra(MediaStore.EXTRA_OUTPUT, fileUri); // set the image file // start the video capture Intent startActivityForResult(intent, CAMERA_CAPTURE_VIDEO_REQUEST_CODE); } /** * Activity result method will be called after closing the camera */ @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { // if the result is capturing Image if (requestCode == CAMERA_CAPTURE_IMAGE_REQUEST_CODE) { if (resultCode == RESULT_OK) { // Refreshing the gallery CameraUtils.refreshGallery(getApplicationContext(), imageStoragePath); // successfully captured the image // display it in image view previewCapturedImage(); } else if (resultCode == RESULT_CANCELED) { // user cancelled Image capture Toast.makeText(getApplicationContext(), "User cancelled image capture", Toast.LENGTH_SHORT) .show(); } else { // failed to capture image Toast.makeText(getApplicationContext(), "Sorry! Failed to capture image", Toast.LENGTH_SHORT) .show(); } } else if (requestCode == CAMERA_CAPTURE_VIDEO_REQUEST_CODE) { if (resultCode == RESULT_OK) { // Refreshing the gallery CameraUtils.refreshGallery(getApplicationContext(), imageStoragePath); // video successfully recorded // preview the recorded video previewVideo(); } else if (resultCode == RESULT_CANCELED) { // user cancelled recording Toast.makeText(getApplicationContext(), "User cancelled video recording", Toast.LENGTH_SHORT) .show(); } else { // failed to record video Toast.makeText(getApplicationContext(), "Sorry! Failed to record video", Toast.LENGTH_SHORT) .show(); } } } /** * Display image from gallery */ private void previewCapturedImage() { try { // hide video preview txtDescription.setVisibility(View.GONE); videoPreview.setVisibility(View.GONE); imgPreview.setVisibility(View.VISIBLE); Bitmap bitmap = CameraUtils.optimizeBitmap(BITMAP_SAMPLE_SIZE, imageStoragePath); imgPreview.setImageBitmap(bitmap); } catch (NullPointerException e) { e.printStackTrace(); } } /** * Displaying video in VideoView */ private void previewVideo() { try { // hide image preview txtDescription.setVisibility(View.GONE); imgPreview.setVisibility(View.GONE); videoPreview.setVisibility(View.VISIBLE); videoPreview.setVideoPath(imageStoragePath); // start playing videoPreview.start(); } catch (Exception e) { e.printStackTrace(); } } /** * Alert dialog to navigate to app settings * to enable necessary permissions */ private void showPermissionsAlert() { AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Permissions required!") .setMessage("Camera needs few permissions to work properly. Grant them in settings.") .setPositiveButton("GOTO SETTINGS", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { CameraUtils.openSettings(MainActivity.this); } }) .setNegativeButton("CANCEL", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { } }).show(); } }
Run and test the app on a real device. You can see the app working as shown in the demo screenshots.
One Reply to “Android working with Camera Using Java”